Capture Images & Video in the Flutter App
August 29, 2024
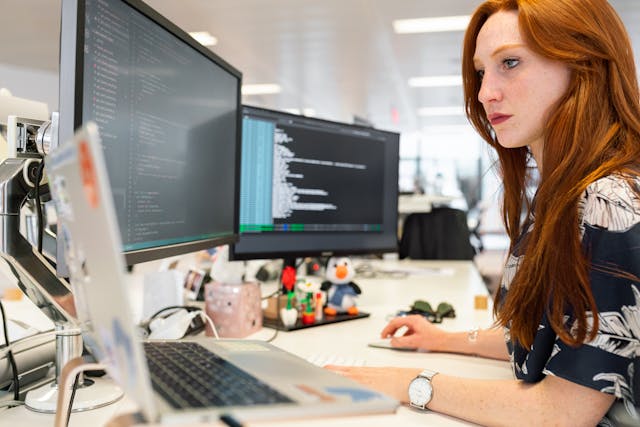
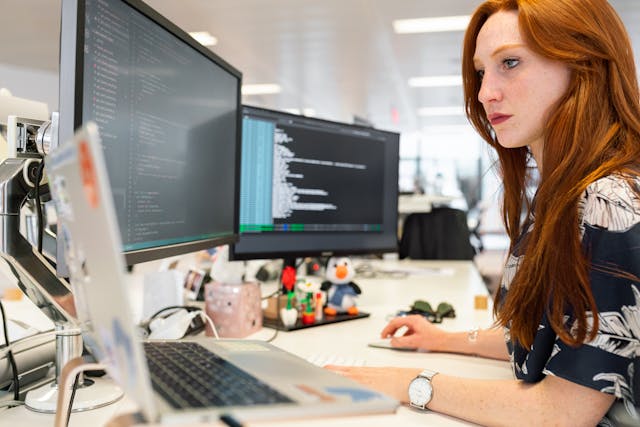
Add Dependencies
First, add the image_picker
package to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
image_picker: ^0.8.7+4
Then, run flutter pub get
to install the package.
Update Android and iOS Configuration
Android
In your android/app/src/main/AndroidManifest.xml
, add the following permissions:
<uses-permission android:name=”android.permission.CAMERA”/> <uses-permission android:name=”android.permission.WRITE_EXTERNAL_STORAGE”/> <uses-permission android:name=”android.permission.READ_EXTERNAL_STORAGE”/>
iOS
In your ios/Runner/Info.plist
, add the following keys:
<key>NSCameraUsageDescription</key> <string>We need camera access to take pictures and videos</string> <key>NSMicrophoneUsageDescription</key> <string>We need microphone access to record videos</string> <key>NSPhotoLibraryUsageDescription</key> <string>We need photo library access to save pictures and videos</string>
3. Create the Flutter App
Here’s a basic Flutter app that allows you to capture images and videos:
import ‘dart:io’;
import ‘package:flutter/material.dart’;
import ‘package:image_picker/image_picker.dart’;
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: CaptureScreen(),
);
}
}
class CaptureScreen extends StatefulWidget {
@override
_CaptureScreenState createState() => _CaptureScreenState();
}
class _CaptureScreenState extends State {
File? _image;
File? _video;
final ImagePicker _picker = ImagePicker();
Future _captureImage() async {
final XFile? pickedFile = await _picker.pickImage(source: ImageSource.camera);
if (pickedFile != null) {
setState(() {
_image = File(pickedFile.path);
});
}
}
Future _captureVideo() async {
final XFile? pickedFile = await _picker.pickVideo(source: ImageSource.camera);
if (pickedFile != null) {
setState(() {
_video = File(pickedFile.path);
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(‘Capture Image/Video’),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: _image != null ? Image.file(_image!) : Text(‘No image selected.’), SizedBox(height: 20), _video != null ? Text(‘Video captured: ${_video!.path}’) : Text(‘No video selected.’), SizedBox(height: 20), ElevatedButton( onPressed: _captureImage, child: Text(‘Capture Image’), ), ElevatedButton( onPressed: _captureVideo, child: Text(‘Capture Video’), ), ,
),
),
);
}
}
Run the Application
Run the app using flutter run
. You should see two buttons to capture an image and video. Once captured, the image will be displayed, and the video path will be shown on the screen.
This is a basic implementation. You can extend it to handle additional features like saving the captured media to a specific location, handling permissions more gracefully, or adding UI improvements.